- Verify AWS Account Permissions
Your IAM user should have the policy with the following permissions:
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"codebuild:StartBuild",
"codebuild:BatchGetBuilds",
"codebuild:BatchGetProjects"
],
"Resource": "arn:aws:codebuild:ap-south-1:ACCOUNT:project/android-sample-app"
},
{
"Effect": "Allow",
"Action": [
"logs:GetLogEvents",
"logs:DescribeLogStreams",
"logs:DescribeLogGroups",
"logs:FilterLogEvents"
],
"Resource": "arn:aws:logs:ap-south-1:ACCOUNT:log-group:/aws/codebuild/android-sample-app:*"
}
]
}
Replace ACCOUNT
with your AWS Account ID.
- Configure AWS Credentials in Jenkins
Open Jenkins Dashboard → Manage Jenkins → Credentials.
Under Global (or your specific folder credentials), add:
- Kind: Secret Text
- ID:
AWS_ACCESS_KEY
→ Paste your AWS Access Key. - ID:
AWS_SECRET_KEY
→ Paste your AWS Secret Key.
- Create Jenkins Pipeline Job
pipeline {
agent { label 'aws-cli-agent' }
environment {
AWS_ACCESS_KEY_ID = credentials('AWS_ACCESS_KEY')
AWS_SECRET_ACCESS_KEY = credentials('AWS_SECRET_KEY')
AWS_REGION="ap-south-1"
PROJECT_NAME = 'android-sample-app'
}
stages {
stage('Build') {
steps {
script {
def exitCode = sh(script: '''
PROJECT_NAME="android-sample-app"
BUILD_ID=$(aws codebuild start-build --project-name $PROJECT_NAME --query 'build.id' --output text)
# Wait for the build to start and fetch the log stream name
LOG_STREAM_NAME=""
while [ -z "$LOG_STREAM_NAME" ]; do
LOG_STREAM_NAME=$(aws codebuild batch-get-builds --ids $BUILD_ID --query 'builds[0].logs.streamName' --output text)
if [ "$LOG_STREAM_NAME" != "None" ]; then
break
fi
sleep 5
done
# Start tailing the logs with the specific log stream name
aws logs tail /aws/codebuild/$PROJECT_NAME --follow --log-stream-names $LOG_STREAM_NAME --format short &
TAIL_PID=$!
while true; do
STATUS=$(aws codebuild batch-get-builds --ids $BUILD_ID --query 'builds[0].buildStatus' --output text)
if [ "$STATUS" != "IN_PROGRESS" ]; then
kill $TAIL_PID
# Set exit code based on build status
case $STATUS in
"SUCCEEDED")
exit 0
;;
"FAILED")
exit 1
;;
"STOPPED")
exit 2
;;
"TIMED_OUT")
exit 3
;;
*)
exit 4
;;
esac
fi
sleep 10
done
''', returnStatus: true)
// Handle build result
if (exitCode == 1 || exitCode == 2 || exitCode == 3) {
currentBuild.result = 'FAILURE'
error "CodeBuild job failed!"
} else {
currentBuild.result = 'SUCCESS'
}
}
}
}
}
}
- Running the Pipeline
Now you can trigger your AWS CodeBuild project in Jenkins pipeline.
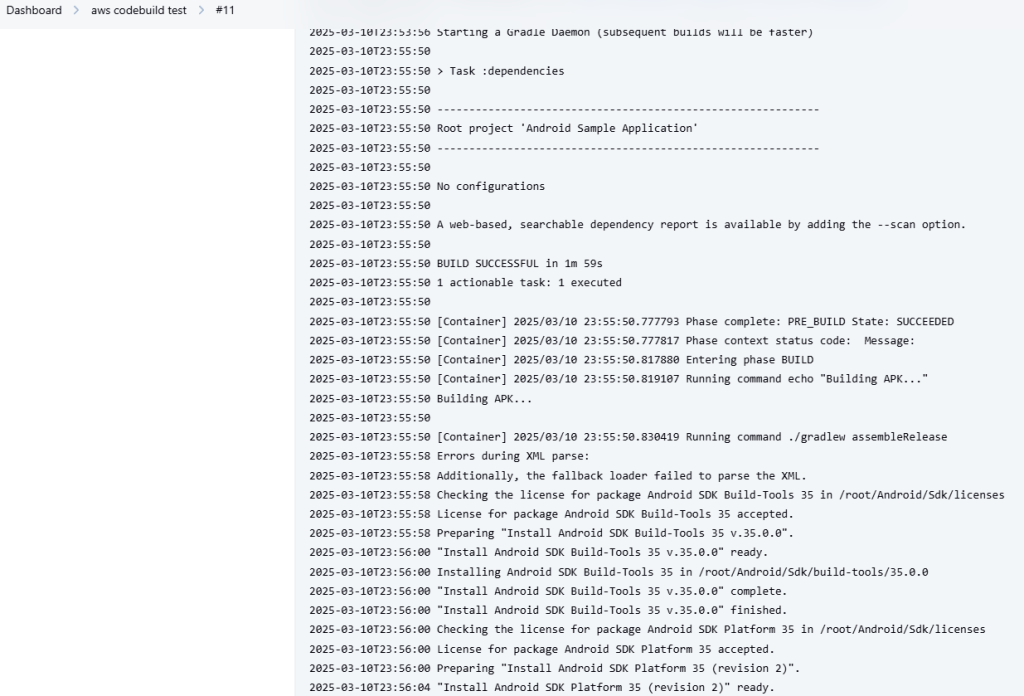